JavaScript Execution
Have you ever wondered how JavaScript operates behind the scenes? This article will take you on a journey through the inner workings of JavaScript, helping you understand concepts like execution context and the call stack, and how these components interact to bring your code to life.
Before diving into JavaScript Execution, it’s important to understand how the browser renders a web page.
Execution Context
In JavaScript, the execution context is the environment where the code is executed. Every time a script is loaded or a function is invoked, a new execution context is created and added to the call stack. But what exactly is an execution context, and how does it define the environment in which our code runs? Now, let’s see how it works.
First we need to understand the Execution Context? When the JavaScript engine processes a script file, it creates an Execution Context, which manages the entire transformation and execution of the code.
There are two types of execution contexts in JavaScript: Global Execution Context and Function Execution Context.
Global Execution Context:
When a JavaScript script initially starts running, the Global Execution Context is created, It representing the global scope.
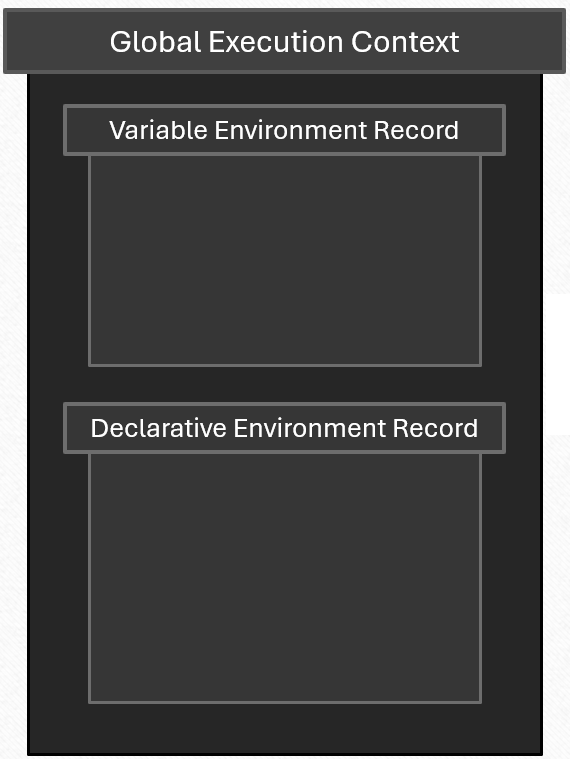
The global execution context has many components, below are some important component.
Variable environment record : The variable environment is a part of the execution context that stores variables declared with var and function declarations. Each execution context, whether global or within a function, has its own variable environment record.
Declarative Environment record : These records are used to store variables declared with let, const, or class declarations.
Function Execution Context:
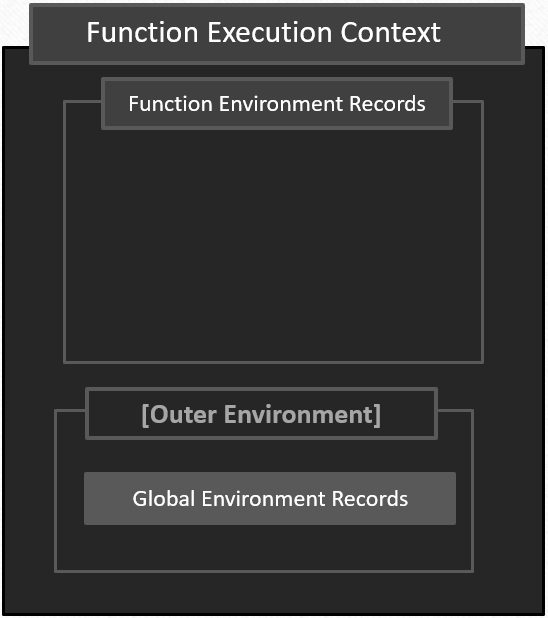
The function execution context is created whenever a function is called, it representing the function’s local scope. Each time a function is called, a new function execution context is created.
Function Environment Records: These are created when a function is called, and they store the function’s arguments, local variables, and inner function declarations.
Scope Chain: Links to the outer environment, allowing access to variables outside the function.
Every execution context goes through two phases Creation Phase and Execution Phase.
Creation Phase: This initial phase involves setting up the environment for the script. In creation phase the memory space is set up for the variable and function.
The creation phase occurs in three stages:
- Creation of the Variable Object (VO): This stores variables and function declarations within the execution context. Function declarations are hoisted, while variables declared with var are hoisted and initialized to undefined.
- Creation of the Scope Chain:This manages lexical scoping, ensuring access to variables and functions from the current and outer scopes.
- Setting the value of the “this” keyword: The ‘this‘ is assigned based on the execution context, referring to the global object in the global context and depending on function invocation in local contexts.
In JavaScript, variables declared with the var keyword are hoisted, while variables declared with let or const keywords are not hoisted. Hoisting applies only to function declarations (statements), not to function expressions.
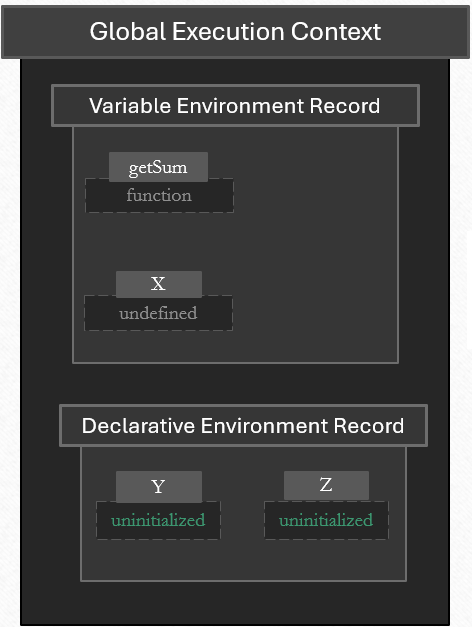
2. Execution Phase: The execution phase in which the execution context is on the call stack and the code is executed. Once the setup is done, the script starts running. The JavaScript engine executes the code within the execution context. It reads and processes statements, evaluates expressions, and performs any necessary function calls.
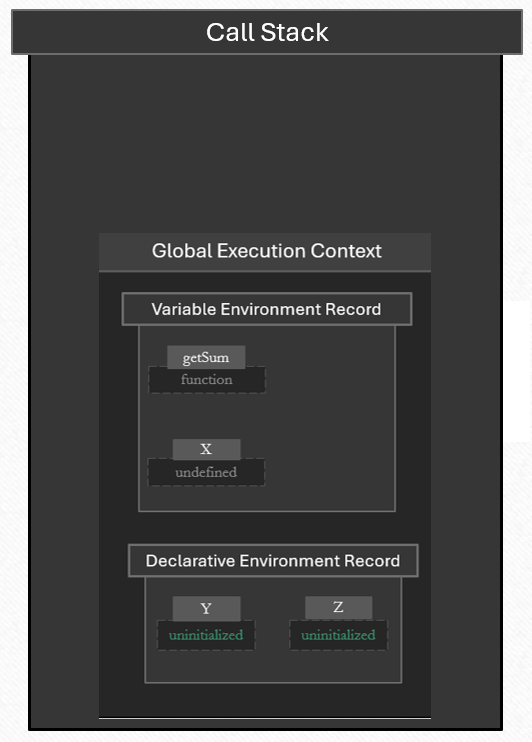
Let’s take an example and see what happens behind the scenes whenever we run this script.
let Y = 5;
var X = 5;
const Z = 10;
function getSum(_x,_y){
var ans = _x +_y;
return ans;
}
var sum = Z+ getSum(X,Y);
Creation Phase: let’s take a look at our script so when parsing this code in the creation phase.
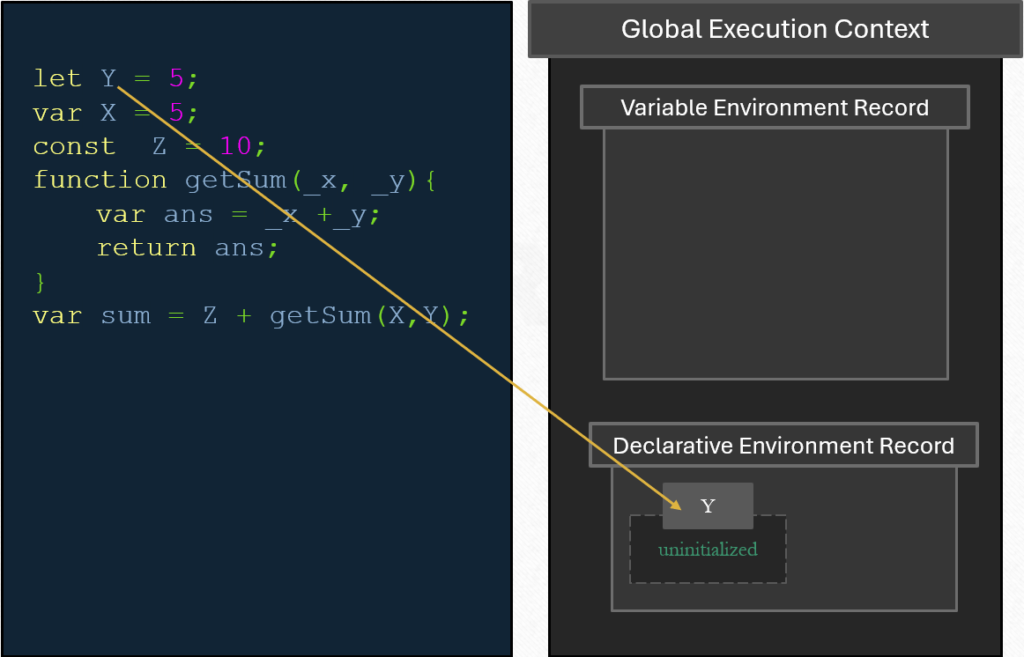
In the creation phase(top to bottom), it first encounters the variable Y and this variable is declared using let, so it will be stored in the Declarative Environment Record associated with the global execution context.
Variables created with the const and let keywords are uninitialized during hoisting, meaning they have memory allocated but no value assigned. They are initialized only during the execution phase of the context, when their values are assigned. The declared is also called the Temporal Dead Zone.
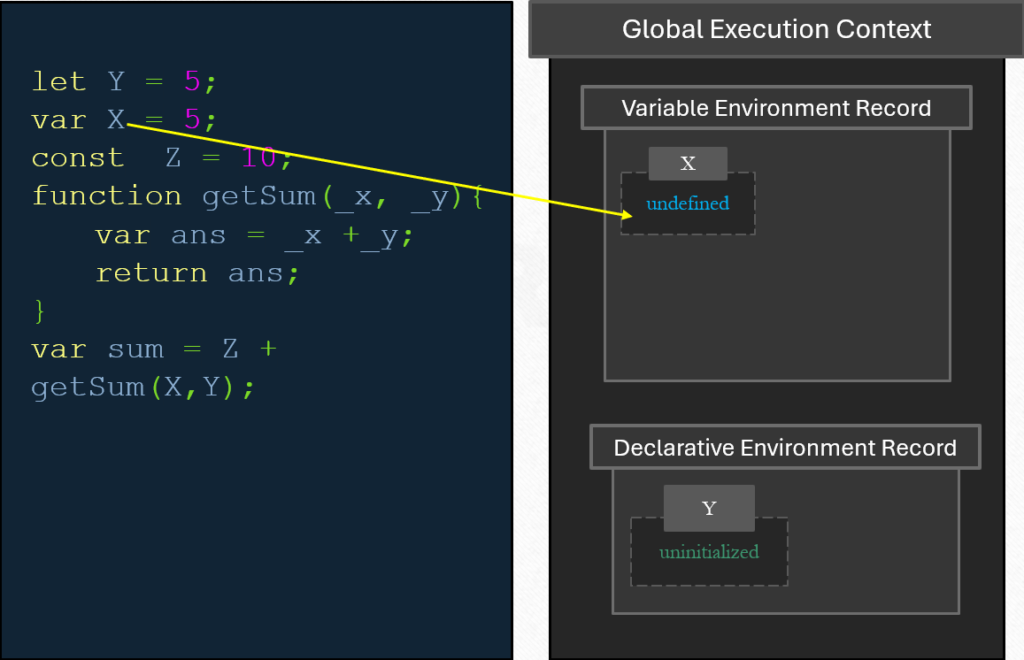
On the 2nd line, X=5, This variable is declared using var, so it will be stored in the Variable Environment Record associated with the global execution context with the value of undefined.
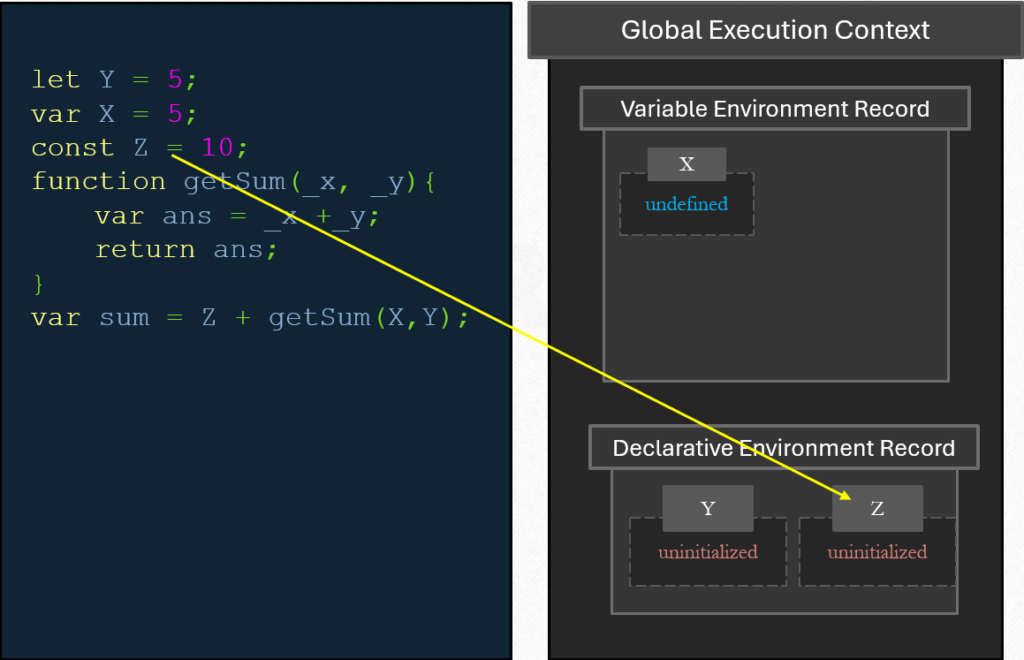
On the 3rd line, Z=10, This variable is declared using const, so it will be stored in the Declarative Environment Record associated with the global execution context.
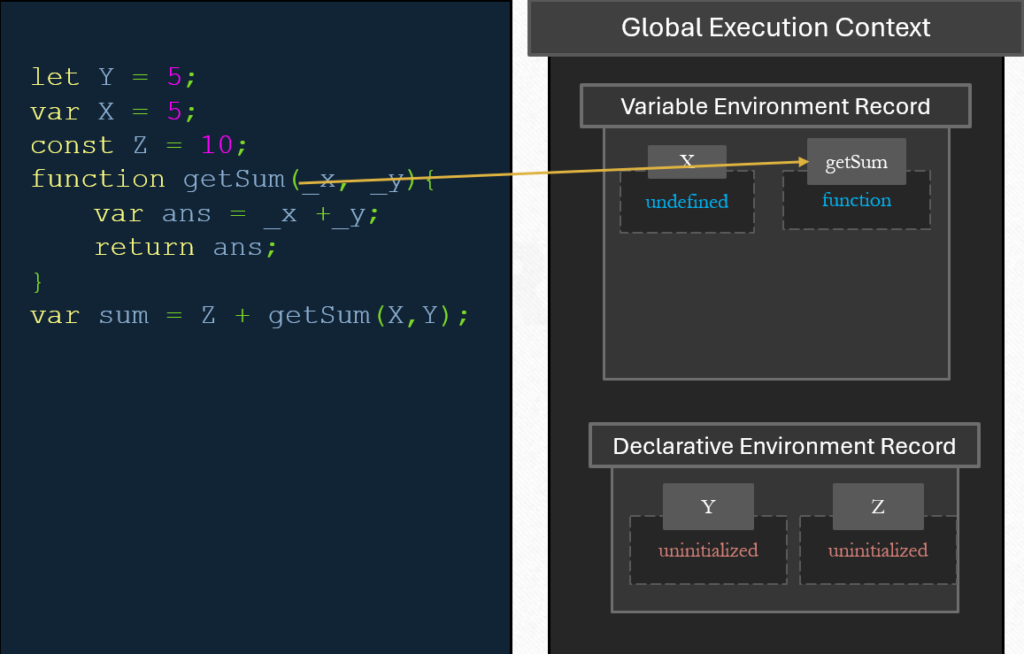
On the next line we have the function getSum.
The getSum function is declared in the global scope, so it will be stored in the Variable Environment Record associated with the global execution context.
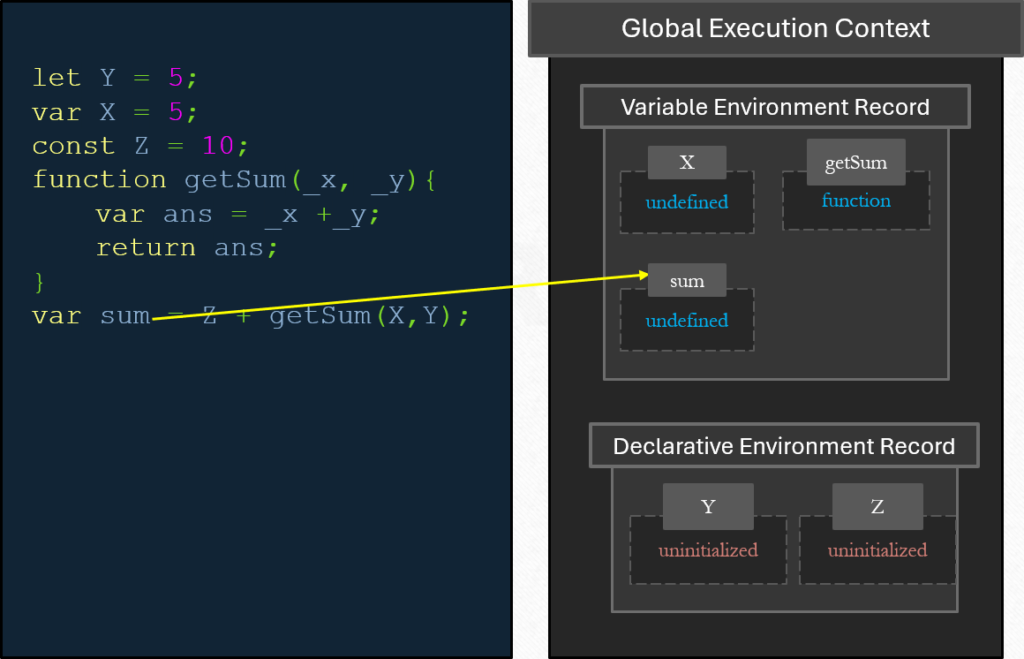
On the last line, sum=Z + getSum(X,Y), This variable is declared using var, so it will be stored in the Variable Environment Record associated with the global execution context with the value of undefined.
There are no other variables or function declarations here, so we move on to the next phase the execution phase.
Execution Phase: The JavaScript engine starts executing the code from top to bottom. This involves running the functions, evaluating expressions, and executing statements.
Variables that were hoisted and initialized to undefined during the creation phase are now assigned their actual values.
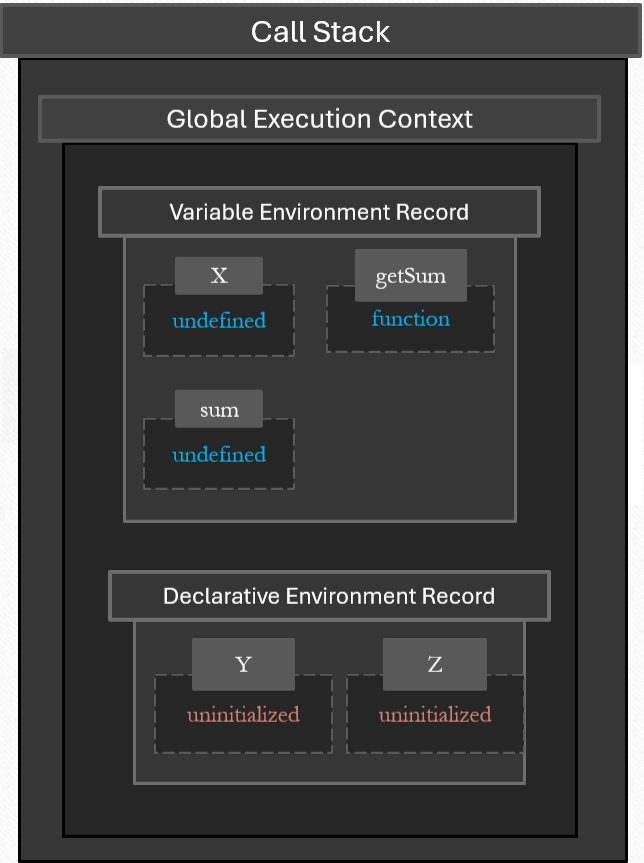
Now the Global execution context is pushed to the Call stack and start execution of code.
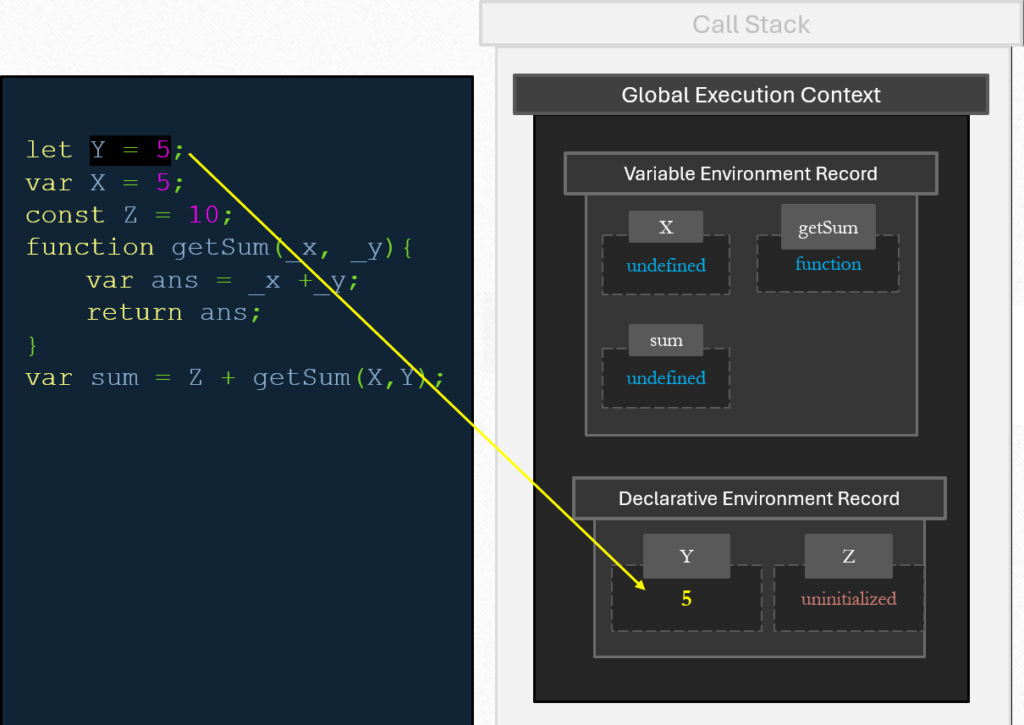
On the first line we have the Y variable so now this variable gets initialized with the value 5.
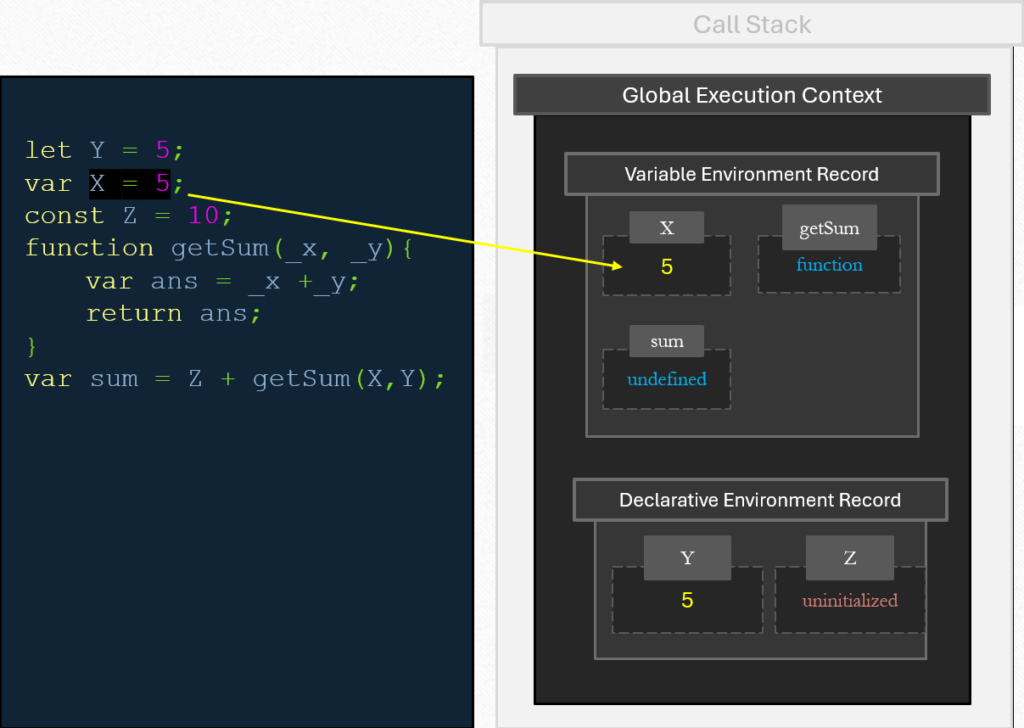
On the 2nd line we have the X variable so now this variable gets initialized with the value 5.
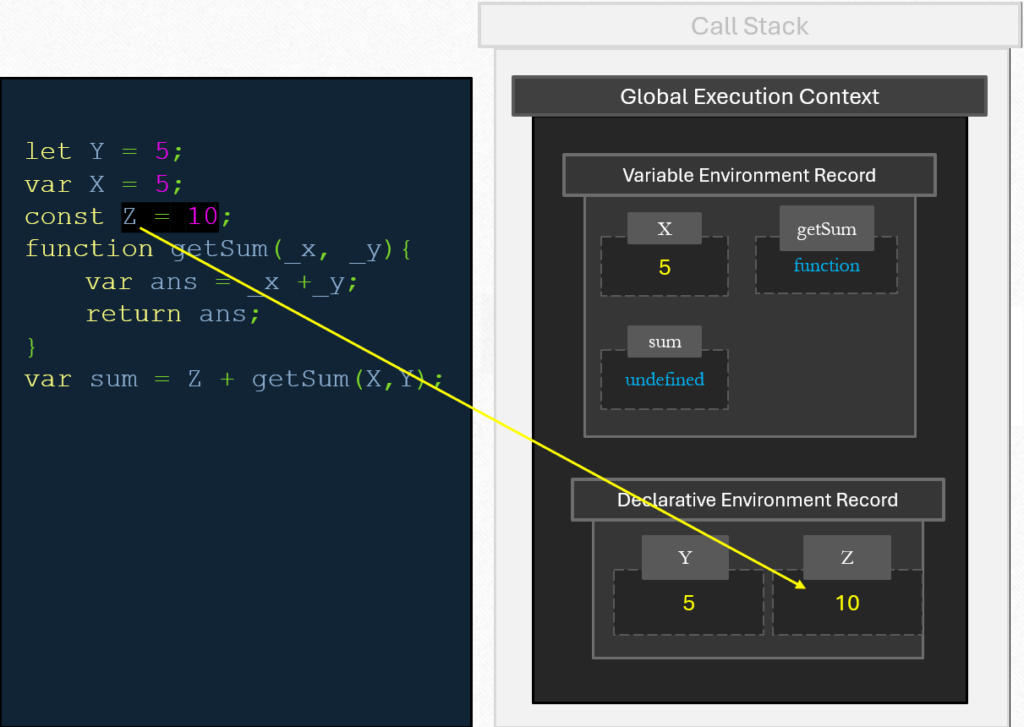
On the 3rd line we have the Z variable so now this variable gets initialized with the value 10.
Then we have the getSum function, but this is already initialized in memory, so nothing gets done here.
on the last line we invoke getSum function, so the call method of the function object is called and this in turn creates a new function execution context and again this execution context goes through two phases, the creation phase and the execution phase. The outer environment points to the global environment record.
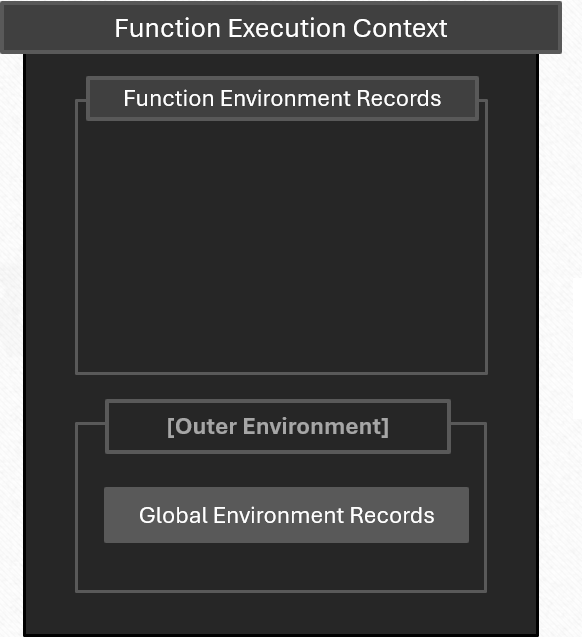
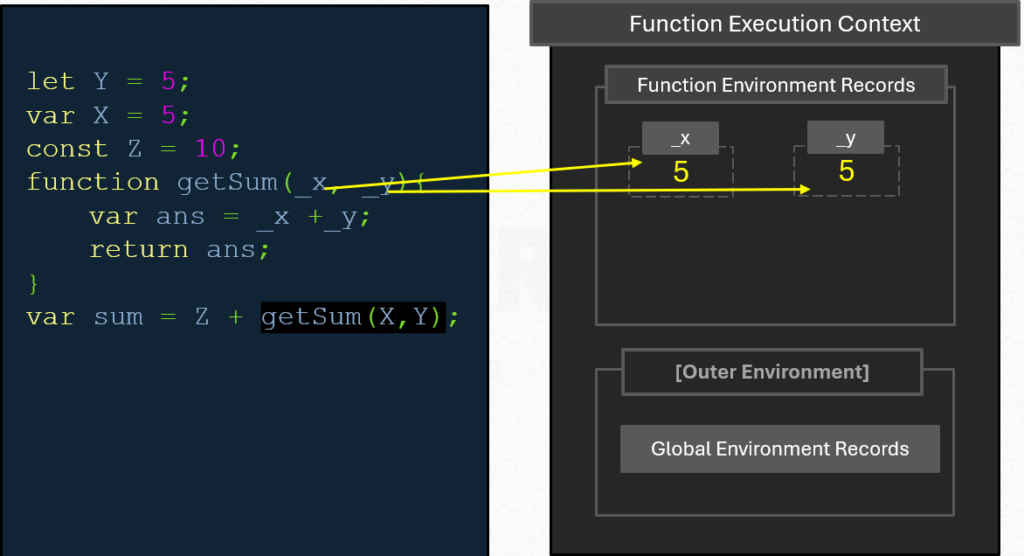
Here we handle function parameters, so in this instance, ‘_X’ and ‘_Y’ are immediately added to the function environment record and immediately initialized with the values 5 and 5, respectively.
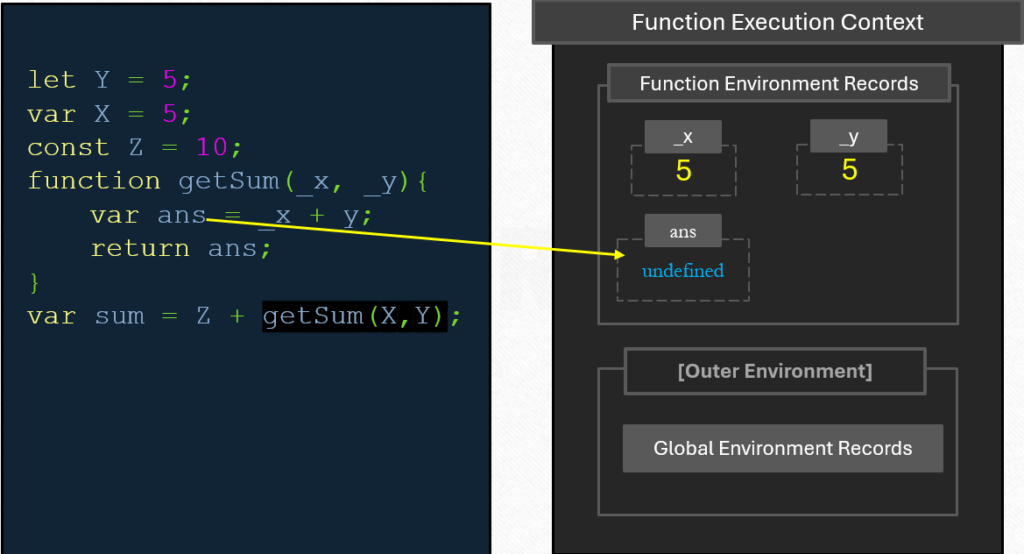
The variables ‘ans’ with the var keyword is added to the function environment and initialized but with the value of undefined.
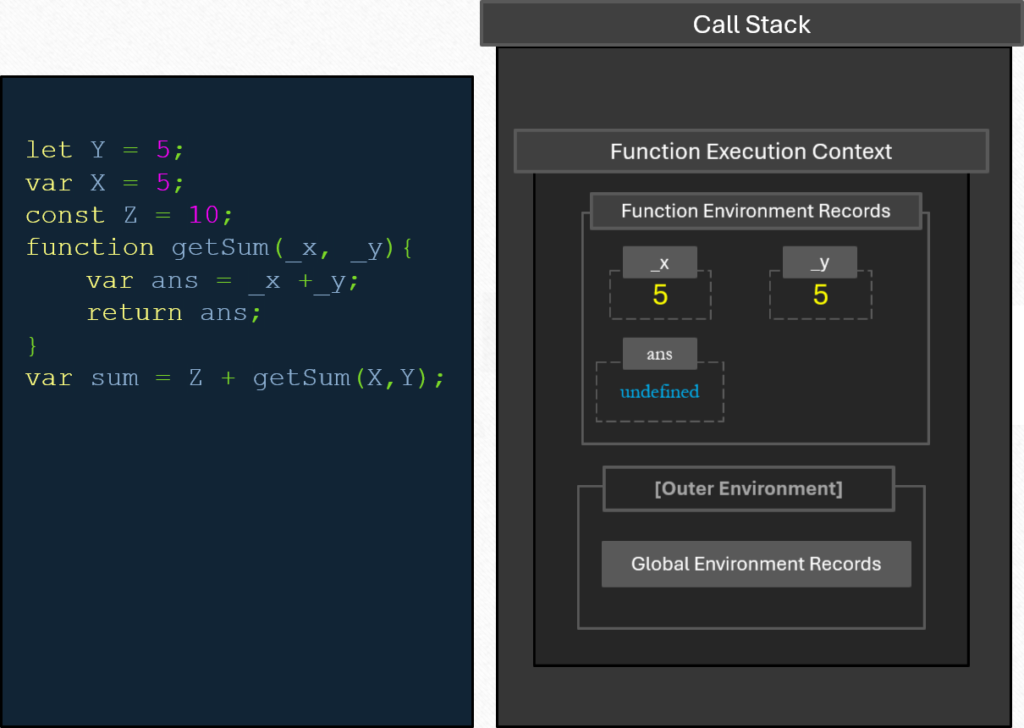
Now that memory has been allocated for the parameters and variables, it’s time for the execution phase so the function execution context is added onto the call stack
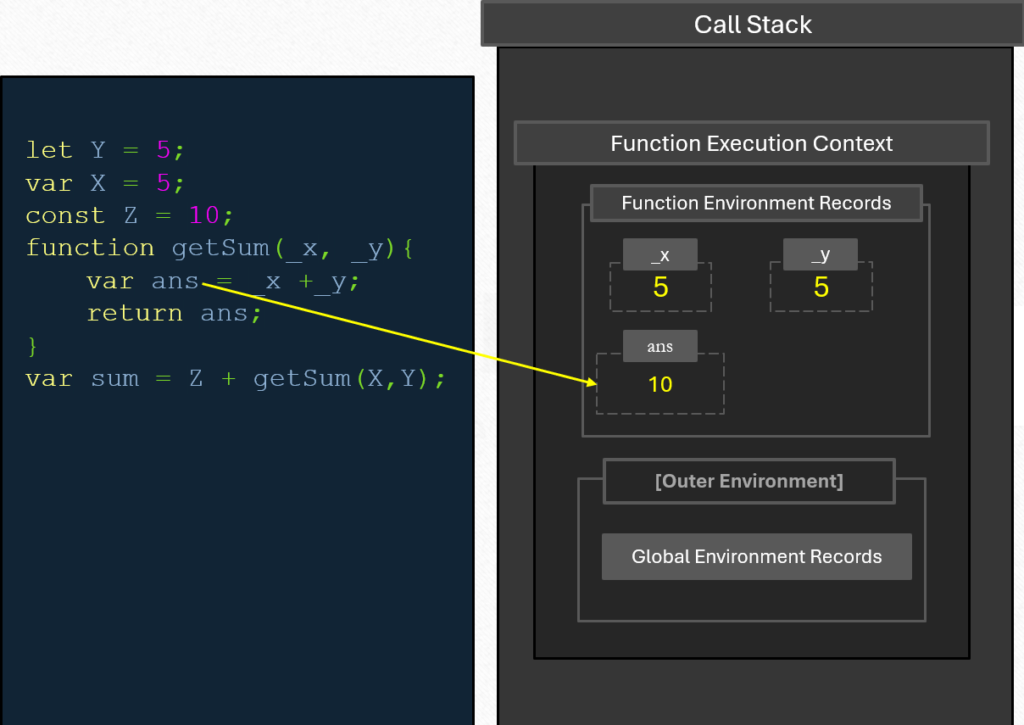
Here, the answer is the sum of 5 and 5, which equals 10. Subsequently, the function returns the value 10.
When the function completes its execution and returns, The function execution context is removed from the call stack. The top most execution context then reverts to the currently active one, which is global context.
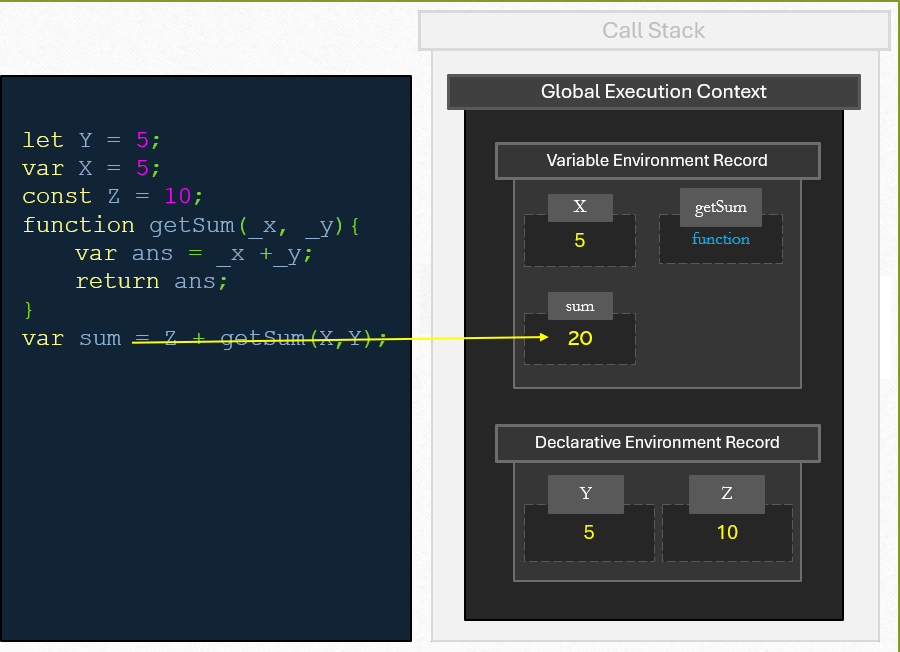
In the end, the value of ‘Z’ (10) is combined with the return value of the getSum function (10), resulting in a total of 20 assigned to the variable ‘sum’.
With no further tasks remaining in our script, the global execution context is also popped off the call stack, signifying the completion of our script.
How the Call Stack Works
The call stack is a fundamental part of how JavaScript executes code, managing the execution contexts in a Last-In-First-Out (LIFO) order.
The call stack operates on the LIFO principle (Last-In-First-Out). When the engine starts executing the script, it creates a global execution context and pushes it onto the stack. Each time a function is invoked, the JavaScript engine creates an execution context for that function, pushes it onto the top of the call stack, and begins executing it.
Once the execution of the current function is complete, the JavaScript engine automatically removes its context from the call stack, returning control to the previous context. Let’s see the following example:
function firstFunction() {
secondFunction();
}
function secondFunction() {
thirdFunction();
}
function thirdFunction() {
console.log('Hello from the third function!');
}
firstFunction();
Call Stack Steps:
- Global Execution Context is created and pushed onto the call stack.
- firstFunction Execution Context is created and pushed onto the stack when firstFunction() is called.
- secondFunction Execution Context is created and pushed onto the stack when secondFunction() is called from within firstFunction.
- thirdFunction Execution Context is created and pushed onto the stack when thirdFunction() is called from within secondFunction.
- Execution of thirdFunction completes, and its context is popped off the stack.
- Execution of secondFunction completes, and its context is popped off the stack.
- Execution of firstFunction completes, and its context is popped off the stack.
- Global Execution Context remains until the script finishes executing.
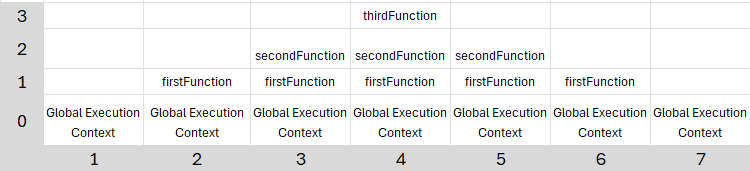
The call stack has a fixed size, which varies depending on the system or browser. If the number of execution contexts exceeds this limit, a stack overflow error occurs. This typically happens with a recursive function.
Some interview questions on JavaScript Execution Phase and Call Stack.
Basic Level
- What is the JavaScript call stack and how does it work?
- Answer: The JavaScript call stack is a data structure that keeps track of the function calls in a program. When a function is invoked, it is added to the top of the stack. When the function completes execution, it is removed from the stack. This process is called “pushing” to the stack and “popping” from the stack.
- Explain what happens during the execution phase of a JavaScript program.
- Answer: During the execution phase, the JavaScript engine executes the code. It creates an execution context for each function call, which includes the scope, variables, and references. The call stack is used to manage the order of execution and track which function is currently running.
- What is the difference between the execution context and the call stack in JavaScript?
- Answer: The execution context is an environment where the JavaScript code is executed and consists of the variable object, scope chain, and
this
keyword. The call stack, on the other hand, is a data structure that keeps track of function calls and manages the execution order of these contexts.
- Answer: The execution context is an environment where the JavaScript code is executed and consists of the variable object, scope chain, and
- Can you explain how JavaScript handles function calls in relation to the call stack?
- Answer: When a function is called, an execution context is created and pushed onto the call stack. The function’s code is executed within this context. If the function calls another function, a new execution context is created and pushed onto the stack. Once a function completes, its context is popped off the stack, and control returns to the previous context.
- What happens if the call stack becomes too large?
- Answer: If the call stack becomes too large, it leads to a stack overflow error. This happens when there are too many nested function calls, typically due to excessive recursion without a base case.
Intermediate Level
- Describe the process of creating an execution context. What are its components?
- Answer: An execution context is created when a function is called and consists of three main components:
- The Variable Object (VO): Stores function arguments, local variables, and inner function declarations.
- Scope Chain: Contains the current variable objects and its parent context’s variable objects.
this
Keyword: Refers to the object context in which the function is executed.
- Answer: An execution context is created when a function is called and consists of three main components:
- How does JavaScript handle asynchronous operations in relation to the call stack?
- Answer: JavaScript uses an event loop to handle asynchronous operations. When an asynchronous operation (e.g., setTimeout, promises, or AJAX calls) is encountered, it is moved to the web APIs, and the main call stack continues executing. Once the asynchronous operation completes, its callback is placed in the callback queue. When the call stack is empty, the event loop pushes the callback onto the call stack to be executed.
- Explain how the call stack interacts with the event loop and callback queue.
- Answer: The call stack executes synchronous code. When the stack is empty, the event loop checks the callback queue for any pending callbacks. If there are callbacks, the event loop pushes them onto the call stack for execution. This process ensures that asynchronous callbacks are executed in a non-blocking manner.
- What is a stack overflow in JavaScript, and how can it be prevented?
- Answer: A stack overflow occurs when there are too many nested function calls, exceeding the stack’s size limit. It can be prevented by avoiding excessive recursion, ensuring base cases are defined in recursive functions, and using iteration instead of recursion when appropriate.
- Provide an example of how a recursive function might affect the call stack.
- Answer: A recursive function, like calculating a factorial, adds a new execution context for each recursive call. If there is no base case or the base case is not reached, it will keep adding contexts, eventually causing a stack overflow .
function factorial(n) {
if (n === 1) return 1;
return n * factorial(n - 1);
}
console.log(factorial(5)); // output 120 Call stack grows with each call
console.log(factorial(50000));
//RangeError: Maximum call stack size exceeded
// at factorial (<anonymous>:2:3)
Advanced Level
How does the JavaScript engine optimize the execution of scripts with respect to the call stack?
Answer: The JavaScript engine optimizes execution by employing Just-In-Time (JIT) compilation, optimizing hot code paths, and using techniques like inlining and tail call optimization to reduce the overhead of function calls and minimize the impact on the call stack.
Explain the concept of tail call optimization and how it affects the call stack.
Answer: Tail call optimization (TCO) is a technique where the JavaScript engine optimizes recursive function calls that are in tail position (i.e., the last action in a function). TCO allows the engine to reuse the current function’s stack frame for the next call, preventing the call stack from growing and avoiding stack overflow.
Discuss the memory management considerations related to the call stack in JavaScript.
Answer: Memory management considerations include avoiding excessive recursion, which can lead to stack overflow and high memory usage. Efficiently managing function calls and leveraging iterative solutions over recursive ones can help. Modern JavaScript engines also use garbage collection to free up memory used by no longer needed execution contexts.
Describe how JavaScript’s event loop mechanism ensures non-blocking I/O operations.
Answer: The event loop mechanism handles asynchronous operations by moving them to web APIs (for I/O operations) and then placing their callbacks in the callback queue once they complete. This allows the call stack to run other synchronous code without blocking. When the stack is empty, the event loop pushes callbacks from the queue onto the stack for execution, ensuring non-blocking I/O.
In a JavaScript engine, what are the performance implications of manipulating the call stack, and how can they be mitigated?
Answer: Performance implications include increased memory usage and potential stack overflow with deep recursion. These can be mitigated by:
- Using iterative approaches where possible.
- Implementing tail call optimization.
- Limiting the depth of recursive calls.
- Using efficient algorithms that minimize the number of function calls.
- Leveraging modern JavaScript features and engine optimizations.